OmqScrollingKeyedTable
The OmqScrollingKeyedTable
can be used to display messages as rows in a java Swing table. The following
is sample code for creating the visual table that will display
messages received from a remote node.
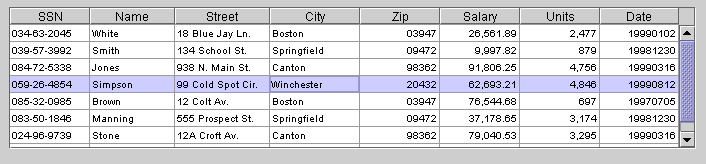
Create a class derived from the TableAttribute class.
Instantiation of the table includes passing a TableAttribute to the constructor
that defines the attributes of the message fields. The following code
is an example of a class derived from the TableAttribute class:
package mypackage;
import osmq.uicontrols.tables.*;
import osmq.datatypes.DataType;
public class MyTableAttrib extends TableAttrib
{
public MyTableAttrib()
{
try {setColumnValues();}
catch (Exception e) {e.printStackTrace();}
}
private void setColumnValues() throws Exception
{
// set the table attributes with 8 visible columns
// ColumnAttrib parms are
// 1) column title
// 2) data type
// 3) column width
addColumnAttrib(new ColumnAttrib("SSN",
DataType.SSN, 11));
addColumnAttrib(new ColumnAttrib("Name",
DataType.STRING, 20));
addColumnAttrib(new ColumnAttrib("Street", DataType.STRING, 30));
addColumnAttrib(new ColumnAttrib("City",
DataType.STRING, 25));
addColumnAttrib(new ColumnAttrib("Zip", DataType.RIGHTALIGN, 8));
addColumnAttrib(new ColumnAttrib("Salary", DataType.CURRENCY, 15));
addColumnAttrib(new ColumnAttrib("Units", DataType.INTEGER, 12));
addColumnAttrib(new ColumnAttrib("Date", DataType.RIGHTALIGN, 10));
}
}
Create the visual table
Create a visual table for displaying the messages. The contstructor takes a class
that identifies the message layout for purposes of mapping it to the visual
grid.
OsmqScrollingKeyedTable mytable = new OsmqScrollingKeyedTable(new MyTableAttrib());
// The messages are keyed for appends and updates
mytable.setUseKeys(true);
Code an onMessage(Message) function
Code the onMessage(Message) function
that will be used to pass messages from the application to the visual
table Messages will be pushed to the onMessage() function once the
network reader is opened.
public void onMessage(Message rm)
{
// Make certain the message is of type DATASET
if(rm.getFormat() != MessageFormat.DATASET)
throw new IllegalArgumentException("Message type is not dataset");
// Pass the message to the visual table component cast as an DataSetMessage,
// where it will be mapped to the screen. Screen mapping will
// involve either an add or update of an existing grid row,
// based on the value of the KEY found in the message header.
mytable.onKeyedDataRow((DataSetMessage) rm);
}
Create, initialize and open a NetReader
Create a network reader server component to retrieve the messages and push them to the onMessage() function.
// create a reader
NetReader reader = new NetReader();
// send messages to this class's onMessage function
reader.setMessageListener(this);
// the reader is in push mode for sending message to this application
reader.setReadMode(NetReader.PUSHMODE);
// Identify the unique service name and local listening port for the network reader
reader.setServiceName("MYSERVICENAME");
reader.setPort(3432);
Open the reader object to begin receiving and processing message
// Start receiving messages from the network reader
_reader.open();
|